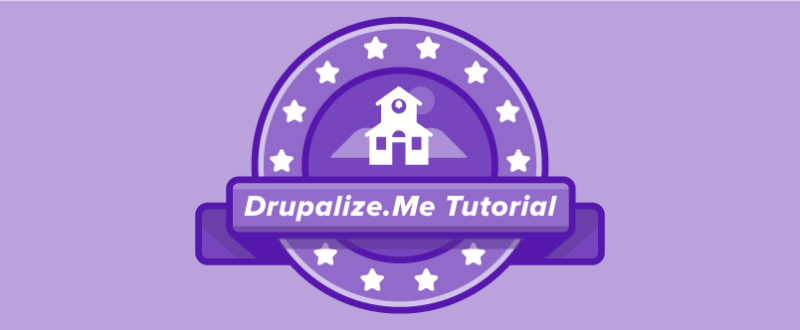
Object-oriented programming (OOP) is way of organizing your code to be more efficient, and it takes advantage of some really nice features in modern versions of PHP. One of the basic concepts of OOP is, not surprisingly, an object. Directly related to working with objects is understanding PHP classes. Drupal 8 is taking big steps to move to an OOP architecture, and so you will get very familiar with both of these as you start to jump into Drupal 8 development.
This tutorial, based on the video Create a Basic PHP Class, will explain what a class is, show you how to set up a class, and look at a PHP object. By the end of this tutorial, you will be able to create a class, an object, add a property to your object, and set the value of the property inside the class.
Before you get started you should be familiar with PHP development generally, and be comfortable with concepts like variables, functions, and arrays. You can take a look at our PHP for Beginners video tutorials if you need to get up to speed. You'll also need to have a computer running PHP with a web server if you want to see the file in action. You can use the built-in PHP server (video tutorial) or you can set up an AMP stack (Apache, MySQL, PHP). Use whichever you prefer. You can grab the base project files for the OOP series to use, or just create your own.
Classes, Objects, and Properties
Before we create a class and then instantiate an object from it, let's do a quick review of what those are. You can think of a class as a template for making objects. Objects are bundles of information and actions you can take with that information. An object can have properties, which are various characteristics, like a name, or a color. They look very much like a variable. It can also have methods, which are standard functions for things the object can do. When you define a class for something, you set up a template that contains all of the standard properties and methods. You then instantiate an object from that class, and fill in the details for this particular object.
Here is a more concrete example, which we'll be using in this tutorial. Let's say that you are working on a starbase and you are responsible for keeping track of all the ships there. They are all generically "ships" but they each have different characteristics, or properties, which distinguish them from each other. To keep things consistent and organized, we'll create a spreadsheet for all the information we need to track, listing all the properties we will need to fill in. This is our class; our template. When a new ship arrives, we open our template and instantiate a new ship document, which is our object. We will fill in all the details specific to this ship: name, weight, weaponry, etc.
Now you could do similar things with procedural PHP using arrays, variables, and functions for sure. Using classes and objects though introduces more rigor to the data and how it gets used, which makes it more consistent and reusable code. If you think of an array as the equivalent to an object, you can define keys and vlaues in the array, much like properties, which can accomplish the same goal. The problem with arrays though is that anyone could put in whatever key they wanted there. This is more like a spreadsheet template that just has blank spaces you need to fill in. I could decide I wanted to define one ship's color, but skip that for another ship and instead write down it's length in centimeters. Objects let us define the template more clearly, and gives us the tools to enforce it.
Hands-on
Once you have a your PHP ready to go, we're going to create a new file and start adding our PHP to it. In your project create a new PHP file. The example project we are working with lets us create different space ships and then have them battle each other. We're going to use a class to create ship objects and be able to define the properties of each ship. To go along with the example project files, I'm going to call my new PHP file play.php, but you can call it what you like.
1. Creating a class is as simple as typing the class
keyword and giving it a name, along with some curly braces, which will contain the default property list for all ships. I'll name my class Ship.
class Ship {
// Object properties will go here.
}
2. To create an actual ship object, we need to instantiate the class. You do this by creating a new variable and then using the `new` keyword with our class name.
$myShip = new Ship();
3. So far we don't have any properties defined for our ship, so let's add a property to our class. Within the class' curly braces, add the `public` keyword followed by a name property.
class Ship {
public $name;
}
4. Now we can add this property information to the new ship object we've created. We're going to add to the existing object by using the "arrow" (`->`) operator.
$myShip->name = 'Jedi Starship';
If you keep reloading this PHP file in your browser, you're not going to see anything at all, but you now have an object with a property that you can access. Let's print out our ship name to the browser.
5. We can use a simple echo or print statement with our object property. Just like a variable that has been defined, we print it out the same way.
print('Ship Name: ' . $myShip->name);
6. Go to the file in your browser and reload the page. You should see the text displaying your ship name.
Our final code for defining the class, creating a ship object, adding a name property, and then printing it to the screen is:
// Define our class.
class Ship {
// Define the properties.
public $name;
}
// Instantiate a new Ship object.
$myShip = new Ship();
// Define the name property for this Ship object.
$myShip->name = 'Jedi Starship';
// Print the ship name to the page.
print('Ship Name: ' . $myShip->name);
You can download the completed play.php file below. Note that the file has .txt appended to it, so you will need to remove that before it will work in your browser.
Summary
In this brief tutorial we've explained the OOP concepts of classes and objects. To put it into practice, we created a new file, made our class, and then instantiated a ship object with a name property. There is a lot more to classes and objects than this very simple introduction. We've just looked at the very basic concepts here, and you'll want to also explore the world of methods, public versus private, and many more features. That belongs in another tutorial though. If you'd like to dive into these right away, you can check out the rest of our Introduction to Object-oriented PHP video series.
Add new comment