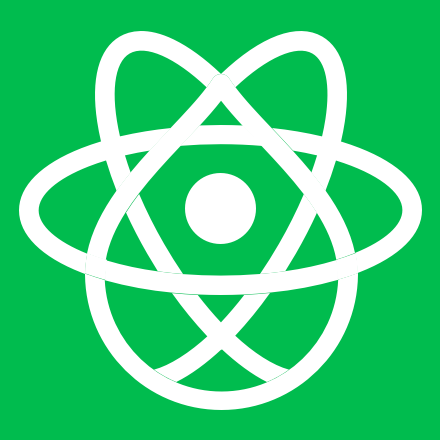
In order for our React code to list content from Drupal we'll need to enable the Drupal core JSON:API module, and then use fetch()
in our React component to retrieve the desired data. This technique works for both React code embedded in a Drupal theme or module, and React code that is part of a fully decoupled application. We'll discuss the differences between those styles as well.
In this tutorial we'll:
- Use
fetch()
to bring data from Drupal into React - Parse the data using ES6 array functions to find just the bits of data we need
- Combine multiple React components together to render a list of articles retrieved from Drupal
By the end of this tutorial, you should have a better understanding of how data from a Drupal API gets incorporated into a React application.
To perform create, update, and delete (CRUD) operations with Drupal core's JSON:API via React there are a few things you'll need to understand. First, how to format the POST
, PATCH
, and DELETE
requests necessary to add, edit, and delete Drupal entities. Next, how to handle authentication, and cross-site request forgery (CSRF) tokens. Over the next few tutorials we will create a simple but powerful React application that can add, edit, and delete Drupal node content.
This tutorial contains:
- An overview of the application we're building
- Information about making secure authenticated requests to Drupal's JSON:API
- An overview of the API requests we'll use to create, update, and delete nodes
By the end of this tutorial, you should have a picture of the application we're going to build, and know how to make the API requests we'll use in our application.
Using React we can do more than just list content. By using the POST, PATCH, and PUT methods of Drupal core's JSON:API web service we can also add, update, and delete, content entities. To demonstrate how this works we'll create a small React application with a form that lets you add, edit, and delete article nodes.
In this tutorial we'll:
- Learn how to handle user authentication and CSRF tokens in a React application
- Create a single React component that outputs a form to add or edit content
- Create a wrapper around the JavaScript
fetch
API to assist in dealing with requests to Drupal's JSON:API
By the end of this tutorial you should know how to create, edit, and delete content in a Drupal site using a React application.
Now that we know how to build a working application in React and embed the application in Drupal, let's make a stand-alone version of our application which can be used outside of the context of a Drupal module or theme. In the next few tutorials we'll look at how to create a fully decoupled React application whose only interactions with Drupal happen via API requests.
In this tutorial we'll:
- Introduce differences we need to account for in a fully decoupled application
- Provide an example of what the final project will look like
By the end of this tutorial you should have a better understanding of what we're trying to create in the rest of this series.
Using create-react-app we can scaffold a stand-alone React application with boilerplate configuration and organization already in place. It's a great way to get started using React, as well as the ecosystem of associated tools like Webpack, Jest, and Babel. After creating the scaffolding, we'll port the code we wrote in previous tutorials to the new structure.
In this tutorial we'll:
- Use
create-react-app
to scaffold a new React project - Refactor existing code into the organizational structure used by
create-react-app
- Confirm that our code runs
By the end of this tutorial, you should know what create-react-app
is and how to get started using it.
When you create a fully decoupled application, the code in your application can't rely on things like the fetch()
function's same-origin
policy and the browser's use of cookies to authenticate requests. Instead, you need to use alternative methods like OAuth or JSON Web Tokens (JWTs).
We'll focus on setting up and using Drupal as an OAuth provider, and allowing a decoupled application to authenticate users via OAuth. This same technique applies just as well if you want to use JWTs.
In this tutorial we'll:
- Install the Simple OAuth Drupal module, and configure it to work with a password grant flow to allow our code to exchange a username and password for an access token
- Demonstrate how to retrieve and use an OAuth access token to make authenticated requests
By the end of this tutorial you should know how to install and configure the Simple OAuth module and make authenticated API requests using an OAuth password grant flow.
To perform POST, PUT, and DELETE operations to Drupal's JSON:API via a decoupled React application we need to use an OAuth access token. This requires first fetching the access token from Drupal, and then including it in the HTTP Authorization
header of all future requests. We'll also need to handle the situation where our access token has expired, and we need to get a new one using refresh token.
To update our example application we need to first write some JavaScript code to manage the OAuth tokens. Then we'll update our existing React components to use that new code.
In this tutorial we'll:
- Write code to exchange a username and password for an OAuth access token using the password grant flow
- Create a wrapper for the JavaScript
fetch()
that handles inserting the appropriate HTTPAuthorization
headers - Update existing code that calls the Drupal JSON:API to use the new code
By the end of this tutorial you'll be able to use OAuth access tokens to make authenticated requests in a React application using fetch
.
React Fast Refresh (formerly Hot Module Replacement) is a technique for using Webpack to update the code that your browser renders without requiring a page refresh. With fast refresh configured it's possible to edit your JavaScript (and CSS) files in your IDE and have the browser update the page without requiring a refresh, allowing you to effectively see the results of your changes in near real time. If you're editing JavaScript or CSS it's amazing. And, it's one of the reasons people love the developer experience of working with React so much.
If you're writing React code as part of a Drupal theme it's possible configure fast refresh to work with Drupal. Doing so will allow live reloading of any JavaScript and CSS processed by Webpack.
In this tutorial we'll:
- Walk through configuring Webpack and fast refresh for a Drupal theme
- Add an
npm run start:hmr
command that will start the webpack-dev-server in hot mode - Configure the webpack-dev-server to proxy requests to Drupal so we can view our normal Drupal pages
By the end of this tutorial you should know how to configure Webpack to allow the use of React's fast refresh feature to your Drupal theme and preview changes to your React code in real time.
Every theme can contain an optional THEMENAME.theme file. This file contains additional business logic written in PHP and is primarily used for manipulation of the variables available for a template file, and suggesting alternative candidate template file names. Themes can also use this file to implement some, but not all, of the hooks invoked by Drupal modules.
In this tutorial we'll learn:
- The use case for THEMENAME.theme files, and where to find them
- The different types of functions and hooks you can implement in a THEMENAME.theme file
By the end of this tutorial you should be able to know how to start adding PHP logic to your custom theme.
Themes and modules can alter the list of theme hook suggestions in order to add new ones, remove existing ones, or reorder the list. This powerful feature allows for the definition of custom logic in your application that can tell Drupal to use different templates based on your own unique needs. You might for example; use a different page template for authenticated users, or a custom block template for someone's birthday.
In this tutorial we'll cover:
- Adding new theme hook suggestions from a theme using
hook_theme_suggestions_HOOK_alter()
- Altering the list of theme hook suggestions
- Removing theme hook suggestions
- Reordering the list of theme hook suggestions
Underscore.js is a very small library which provides several utility functions and helpers to make working with JavaScript a little bit easier. In this tutorial we'll take a look at a part of the library, learn where the full library is documented, and see how we can make use of Underscore.js in a custom block on our Drupal site.
Make your theme a subtheme of a base theme, allowing it to inherit all the base theme's templates and other properties. When creating Drupal themes it is common to use the Classy theme provided with Drupal core as a base theme to jumpstart your development.
In this tutorial we'll learn how to:
- Use the
base theme
key in our theme's THEMENAME.info.yml file - Make our Ice Cream theme inherit from the Classy theme, or any other theme
By the end of this tutorial you should be able to tell Drupal that your theme is a child of another theme and should inherit all of the parent theme's features.
In Drupal, whenever we output markup it's best practice to use a Twig template or a theme function. But whenever you need to output DOM elements within JavaScript the best practice is to use the Drupal.theme
function. This function ensures that the output can be overridden just like the HTML output by Twig. This tutorial covers how to use the Drupal.theme
function in your JavaScript when inserting DOM elements, as well as how to replace the markup output by other JavaScript code that is using the Drupal.theme
function.
ESLint is the linting tool of choice for JavaScript in Drupal. In this tutorial we’ll show how to install the ESLint application and then use it to verify that your JavaScript files are meeting the Drupal coding standards.
Drupal (as of version 8.4) has adopted the Airbnb JavaScript coding standards. In this tutorial, we'll walk through how to install the necessary package dependencies to run eslint on JavaScript files within your Drupal site.
An asset library is a bundle of CSS and/or JavaScript files that work together to provide a style and functionality for a specific component. They are frequently used to isolate the functionality and styling of a specific component, like the tabs displayed at the top of each node, into a reusable library. If you want to include CSS and/or JavaScript in your Drupal theme or module you'll need to declare an asset library that tells Drupal about the existence, and location, of those files. And then attach that library to a page, or specific element, so that it gets loaded when needed.
In this tutorial we’ll:
- Define what an asset library is.
- Explain why asset libraries are used to include JavaScript and CSS files.
- Look at some example asset library definitions.
By the end of this tutorial you should be able to define what asset libraries are, and when you'll need to create one.
Preprocess functions allow Drupal themes to manipulate the variables that are used in Twig template files by using PHP functions to preprocess data before it is exposed to each template. All of the dynamic content available to theme developers within a Twig template file is exposed through a preprocess function. Understanding how preprocess functions work, and the role they play, is important for both module developers and theme developers.
In this tutorial we'll learn:
- What preprocess functions are and how they work
- The use case for preprocess functions
- The order of execution for preprocess functions
By the end of this tutorial you should be able to explain what preprocess functions are and the role they play in a Drupal theme.
Template files are responsible for the HTML markup of every page generated by Drupal. Any file ending with the .html.twig extension is a template file. These files are composed of standard HTML markup as well as tokens used by the Twig template engine to represent dynamic content that will be substituted into the HTML markup when the template is used. As a theme developer, you'll work with this a lot.
In this tutorial we’re going to learn about:
- What template files are, and how they fit into the big picture of creating a theme
- How template files are used in order to allow theme developers to modify the HTML markup output by Drupal
- Naming conventions for, and specificity of, template files
What Is a Theme?
FreeThemes are the part of Drupal that you, and anyone else visiting your Drupal powered application, see when they view any page in their browser. You can think of a theme as a layer, kind of like a screen, that exists between your Drupal content and the users of your site. Whenever a page is requested Drupal does the work of assembling the content to display into structured data which is then handed off to the presentation layer to determine how to visually represent the data provided.
Drupal themes are created by front-end developer. Frequently referred to as themers, or theme developers. Themes consist of standard web assets like CSS, JavaScript, and images, combined with Drupal-specific templates for generating HTML markup, and YAML files for telling Drupal about the file and features that make up each individual theme.
In this tutorial we'll:
- Explain what a Drupal theme is.
- Explain the role of a Drupal themer in the process of building a Drupal site.
- Get a high level overview of the types of files/code that themes are made of.
By the end of this tutorial you should be able to explain what a Drupal theme is, and the kind of work a Drupal theme developer will be expected to do.
Maybe you've heard of anonymous closures but you're not quite sure how they apply in Drupal, or why using them is considered a best-practice. Anonymous closures allow you to avoid accidentally clashing with anything in the global scope, as well as to alias the jQuery object to the more commonly used $
. This is necessary because Drupal runs jQuery in no-conflict mode. This tutorial will look at the syntax used for placing your custom JavaScript code inside an anonymous closure, and why it's a good idea to do so.
In this tutorial we'll:
- Explain what a closure is (briefly), and what immediately invoked function expressions are
- Show how typically Drupal JavaScript gets wrapped in a closure
- Provide a copy/paste example you can use in your own code
By the end of this tutorial you should be able to explain what an anonymous closure is, and how to use one in your custom JavaScript for Drupal.
Like most output in Drupal, Views relies on Twig templates for a significant amount of its rendering. In this tutorial we'll identify where you can find the default Views templates within your file system, what the common templates are for, and how to name your templates so that they are applied to specific views.
By the end of this tutorial, you should be able to:
- Identify where to find default views templates
- Understand which templates apply to what part of a view
- Get a sense of the template suggestions and how to use them to limit where your custom templates are applied
- Identify a view's machine name
- Identify a display's machine name
- Identify a field's machine name