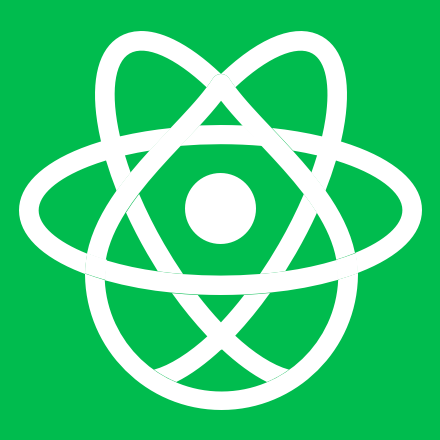
Themes and modules can alter the list of theme hook suggestions in order to add new ones, remove existing ones, or reorder the list. This powerful feature allows for the definition of custom logic in your application that can tell Drupal to use different templates based on your own unique needs. You might for example; use a different page template for authenticated users, or a custom block template for someone's birthday.
In this tutorial we'll cover:
- Adding new theme hook suggestions from a theme using
hook_theme_suggestions_HOOK_alter()
- Altering the list of theme hook suggestions
- Removing theme hook suggestions
- Reordering the list of theme hook suggestions
In Drupal's Appearance UI, all themes get a theme settings form. As a theme developer, you can customize the theme settings form, enabling site administrators to have more control over the appearance of the theme.
In this tutorial, we'll show you how to add admin-configurable settings to your theme. In the process of doing this, we'll use a variety of theme and module development skills and assume you have some familiarity with using Drupal's Form API, Configuration API, and theme system (see Prerequisites).
By the end of this tutorial, you will be able to provide custom theme settings that an administrator can use to modify the appearance of the theme.
Drupal media entities are fieldable entities, which means that you can add any custom fields you want to your Media types. These fields can be used for collecting additional metadata about a resource, categorizing and organizing resources so they're easier to find in a large media library, or for displaying information like a photo credit or transcript for a video. The possibilities are endless once you know how to add, and optionally display, fields in Drupal.
Some example use cases for adding fields to Media types:
- Collect, and display, a credit to go along with a photo. This could also be a date, a location, or any other metadata.
- Store resource width and height dimensions as custom fields so they can be referenced by display logic in the theme layer.
- Use Taxonomy reference fields to add tags or categories to help keep a large library organized.
In this tutorial we'll:
- Learn how to add fields to a Media type
- Verify our new custom field is working
By the end of this tutorial you'll know how to add custom fields to any Media type.
Custom themes in Drupal must be configured to inherit settings, templates, and other assets from a parent theme. Which base theme you use is configurable. This allows theme developers to use a different set of markup as the starting point for their theme, organize various theme assets into a more maintainable structure, and more. All of this is made possible because of how Drupal's theme layer uses a chain of inheritance when assembling all the parts of a theme.
Base themes are also a powerful way to encapsulate standards and best practices into a reusable code base. You'll find dozens of contributed base themes on Drupal.org that can serve as a great starting point, especially if you're planning to work with an existing design framework like Bootstrap or Susy Grids. Or if you want to leverage modern JavaScript bundling without setting up Webpack on your own.
In this tutorial we'll:
- Learn what base themes and subthemes are
- Look at a few examples of template inheritance and how that works
- Discuss some use cases for theme inheritance
By the end of this tutorial you'll know how to declare the base theme that your theme builds upon.
Writing a React application requires including the React JavaScript library in the page, writing some React-specific JavaScript, and then binding it to a specific DOM element on the page. You may also want to include existing packages from the npm ecosystem, and use modern JavaScript (ES6+) features, which necessitates setting up a build toolchain for your JavaScript using a tool like Webpack or Parcel.
There are a lot of different ways you could go about setting this all up. Do you add React via a theme or a module? Do you need a build tool? Should you use Webpack, or Babel, or Parcel, or something else? While we can't possibly cover all the different approaches, we can help you figure out what is required, and you can adapt our suggestions to meet your needs.
In this tutorial we'll:
- Create a new custom theme with the required build tools to develop React applications
- Add a DOM element for our React application to bind to
- Create a "Hello, World" React component to verify everything is working
By the end of this tutorial you'll know how to configure everything necessary to start writing React within a Drupal theme.
Every theme can contain an optional THEMENAME.theme file. This file contains additional business logic written in PHP and is primarily used for manipulation of the variables available for a template file, and suggesting alternative candidate template file names. Themes can also use this file to implement some, but not all, of the hooks invoked by Drupal modules.
In this tutorial we'll learn:
- The use case for THEMENAME.theme files, and where to find them
- The different types of functions and hooks you can implement in a THEMENAME.theme file
By the end of this tutorial you should be able to know how to start adding PHP logic to your custom theme.
React and Drupal can be used together in two different ways: fully decoupled, also known as headless; or progressively decoupled.
In this tutorial we'll talk about the differences between these two approaches, including:
- Defining what each method refers to
- Considerations regarding hosting, performance, and access
Then we'll link to lots of additional reading materials so you can gain a deeper understanding of the subject.
By the end of this tutorial you should be able to define what decoupled and progressively decoupled mean, and how they differ from one another.
Before we start writing any React code, let's go over some basic concepts and terminology. Throughout this series we'll assume you're familiar with these things. They'll come up again and again as you work on projects that involve React, so it's worth taking the time to learn them.
In this tutorial we'll cover the following at a high level, and provide links to resources:
- Why choose React?
- What are React components?
- What are hooks, state, and JSX?
- The role of build tools when developing React applications
By the end of this tutorial you should have a firm grasp of the fundamental concepts and terminology necessary to start creating React applications.
The Render API consists of a standard format for describing data using structured arrays and a process for converting those arrays into the HTML a user sees when interacting with a Drupal site.
As a theme developer you can extend an existing asset library to include custom CSS and/or JavaScript from your theme. This is useful when you want to add styles or behaviors to components provided by Drupal core or another module.
Sometimes there are CSS or JavaScript asset libraries attached to the page by Drupal core, a contributed module, or another theme, that do something you don't like, and you want to change it or even exclude it all together. There are a couple of different ways that themes can override, alter, or extend, an existing asset library in order to modify the CSS and JavaScript that get attached the page by other code belonging to another theme or module.
In this tutorial we'll learn how to:
- Extend an existing asset library using
libraries-extend
, so that our custom CSS and JavaScript is included whenever that library is used. - Override an existing asset library using
libraries-override
, to alter the definition of the library, and replace or exclude individual assets (or the entire library).
By the end of this tutorial you should be able to use your custom theme to override, extend, or alter any of the asset libraries added to the page by another theme or module.
The Layout Builder Styles module extends the Drupal core Layout Builder UI to add the ability for editors to apply custom CSS classes to the blocks and sections that make up a layout. This gives layout editors more control over the look and feel of elements within a layout. It's especially useful when using Drupal's Layout Builder in conjunction with a design system like Bootstrap, Material UI, or your own predefined utility classes.
The module allows site builders to define new styles. Then, when placing a block into a layout, if there are any styles available for the block type, the user is presented with a select list where they can choose one or more to apply. When a style is applied, any CSS classes associated with the style are added to the markup. Also, a new style-specific theme hook suggestion is added to the block to allow for further customization.
In this tutorial we'll:
- Install and configure the Drupal Layout Builder Styles module
- Learn how to define new styles
- Learn how anyone editing a layout can apply the styles we defined to a block or section in the layout to change the UX
By the end of this tutorial, you should be able to use the Layout Builder Styles module to allow editors to add predefined styles to existing layouts and blocks without writing any code.
Layouts
TopicA layout can describe how various components are arranged on various levels—from an entire page from the header to the footer, to just the “middle” where the dynamic content goes, to individual components. It can apply to templates for managed content or one-off designs for landing pages.
This chapter goes through the process of adding a specific form id to the theme registry with the hook_theme() function, which allows the creation of a new function that targets a specific form. Specifically in this chapter, comment_form is added to the theme registry so that we can create a ninesixtyrobots_comment_form() function in order to add a column to the comment form so that the name and subject fields are displayed side-by-side.
Additional resources
Coding Standards in Drupal
CourseFormatting standards cover things like the use of whitespace, how to format control structures, and other aspects that affect your code's appearance and format.
In this tutorial we’ll talk specifically about standards regarding formatting. This is by no means an exhaustive list of PHP syntax rules, but rather is focused on formatting standards for Drupal.
By the end of this tutorial you'll know about the most common Drupal code formatting standards as well as where to find more information when questions arise.
Translations have their own special functions in both Drupal 7 and 8, and there are some rules for standardizing how they are used that make things clearer for everyone.
In this tutorial we'll look at:
- When to use, and when not to use, translation utilities to output translatable strings
- How placeholders work in translatable strings
- Tips for creating links inside of translatable strings
By the end of this tutorial you should know when, and how, to make strings in your code translatable using Drupal's translation utility functions.
Now that we’re familiar with the default field wrapper markup in Views, let’s explore how to customize this markup. In this tutorial, I will introduce to you a prototype of recent posts that was built using Pattern Lab. We’ll inspect the wrapper markup of two template files that compose this media list. After getting familiar with the prototype markup, we’ll apply that markup, step-by-step, to the fields in our view of recent posts. In doing so, we will use the custom field markup settings for each field and utilize rewrite results and replacement tokens to customize our markup output even further.
Additional resources
Standardized documentation is crucial to a project, whether it is just you or an entire team working on it. In this tutorial we're going to look at:
- Standards for
@docblock
comments - Standards for inline comments
- Why standards for documentation and comments are as important as standards for the rest of your code.
By the end of this tutorial you'll know how to add inline documentation for all the PHP code that you write for Drupal.
Once you know what code standards are and why you should use them, you need to learn how to implement Drupal coding standards in your projects. This tutorial will walk through some of the steps you can take to make this as easy as possible. We'll cover:
- Configuring your editor or IDE to warn you of coding standards violations
- Setting up the Coder module and phpcs to scan and review your code
- Performing team code reviews
By the end of this tutorial you should be able to configure your development environment and implement processes in your workflow that help to ensure your code meets Drupal's coding standards guidelines.