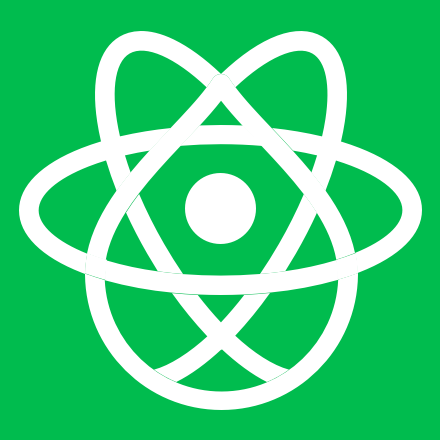
In order for our React code to list content from Drupal we'll need to enable the Drupal core JSON:API module, and then use fetch()
in our React component to retrieve the desired data. This technique works for both React code embedded in a Drupal theme or module, and React code that is part of a fully decoupled application. We'll discuss the differences between those styles as well.
In this tutorial we'll:
- Use
fetch()
to bring data from Drupal into React - Parse the data using ES6 array functions to find just the bits of data we need
- Combine multiple React components together to render a list of articles retrieved from Drupal
By the end of this tutorial, you should have a better understanding of how data from a Drupal API gets incorporated into a React application.
To perform create, update, and delete (CRUD) operations with Drupal core's JSON:API via React there are a few things you'll need to understand. First, how to format the POST
, PATCH
, and DELETE
requests necessary to add, edit, and delete Drupal entities. Next, how to handle authentication, and cross-site request forgery (CSRF) tokens. Over the next few tutorials we will create a simple but powerful React application that can add, edit, and delete Drupal node content.
This tutorial contains:
- An overview of the application we're building
- Information about making secure authenticated requests to Drupal's JSON:API
- An overview of the API requests we'll use to create, update, and delete nodes
By the end of this tutorial, you should have a picture of the application we're going to build, and know how to make the API requests we'll use in our application.
Using React we can do more than just list content. By using the POST, PATCH, and PUT methods of Drupal core's JSON:API web service we can also add, update, and delete, content entities. To demonstrate how this works we'll create a small React application with a form that lets you add, edit, and delete article nodes.
In this tutorial we'll:
- Learn how to handle user authentication and CSRF tokens in a React application
- Create a single React component that outputs a form to add or edit content
- Create a wrapper around the JavaScript
fetch
API to assist in dealing with requests to Drupal's JSON:API
By the end of this tutorial you should know how to create, edit, and delete content in a Drupal site using a React application.
Now that we know how to build a working application in React and embed the application in Drupal, let's make a stand-alone version of our application which can be used outside of the context of a Drupal module or theme. In the next few tutorials we'll look at how to create a fully decoupled React application whose only interactions with Drupal happen via API requests.
In this tutorial we'll:
- Introduce differences we need to account for in a fully decoupled application
- Provide an example of what the final project will look like
By the end of this tutorial you should have a better understanding of what we're trying to create in the rest of this series.
Using create-react-app we can scaffold a stand-alone React application with boilerplate configuration and organization already in place. It's a great way to get started using React, as well as the ecosystem of associated tools like Webpack, Jest, and Babel. After creating the scaffolding, we'll port the code we wrote in previous tutorials to the new structure.
In this tutorial we'll:
- Use
create-react-app
to scaffold a new React project - Refactor existing code into the organizational structure used by
create-react-app
- Confirm that our code runs
By the end of this tutorial, you should know what create-react-app
is and how to get started using it.
When you create a fully decoupled application, the code in your application can't rely on things like the fetch()
function's same-origin
policy and the browser's use of cookies to authenticate requests. Instead, you need to use alternative methods like OAuth or JSON Web Tokens (JWTs).
We'll focus on setting up and using Drupal as an OAuth provider, and allowing a decoupled application to authenticate users via OAuth. This same technique applies just as well if you want to use JWTs.
In this tutorial we'll:
- Install the Simple OAuth Drupal module, and configure it to work with a password grant flow to allow our code to exchange a username and password for an access token
- Demonstrate how to retrieve and use an OAuth access token to make authenticated requests
By the end of this tutorial you should know how to install and configure the Simple OAuth module and make authenticated API requests using an OAuth password grant flow.
To perform POST, PUT, and DELETE operations to Drupal's JSON:API via a decoupled React application we need to use an OAuth access token. This requires first fetching the access token from Drupal, and then including it in the HTTP Authorization
header of all future requests. We'll also need to handle the situation where our access token has expired, and we need to get a new one using refresh token.
To update our example application we need to first write some JavaScript code to manage the OAuth tokens. Then we'll update our existing React components to use that new code.
In this tutorial we'll:
- Write code to exchange a username and password for an OAuth access token using the password grant flow
- Create a wrapper for the JavaScript
fetch()
that handles inserting the appropriate HTTPAuthorization
headers - Update existing code that calls the Drupal JSON:API to use the new code
By the end of this tutorial you'll be able to use OAuth access tokens to make authenticated requests in a React application using fetch
.
React Fast Refresh (formerly Hot Module Replacement) is a technique for using Webpack to update the code that your browser renders without requiring a page refresh. With fast refresh configured it's possible to edit your JavaScript (and CSS) files in your IDE and have the browser update the page without requiring a refresh, allowing you to effectively see the results of your changes in near real time. If you're editing JavaScript or CSS it's amazing. And, it's one of the reasons people love the developer experience of working with React so much.
If you're writing React code as part of a Drupal theme it's possible configure fast refresh to work with Drupal. Doing so will allow live reloading of any JavaScript and CSS processed by Webpack.
In this tutorial we'll:
- Walk through configuring Webpack and fast refresh for a Drupal theme
- Add an
npm run start:hmr
command that will start the webpack-dev-server in hot mode - Configure the webpack-dev-server to proxy requests to Drupal so we can view our normal Drupal pages
By the end of this tutorial you should know how to configure Webpack to allow the use of React's fast refresh feature to your Drupal theme and preview changes to your React code in real time.
Why Solr?
FreeDrupal has long provided a built-in search mechanism, so why do we need anything more? In this tutorial, we introduce Apache Solr, a free and open source search service that has several advantages and features beyond Drupal’s built-in search.
In this tutorial, we'll:
- Define Apache Solr
- Identify Apache Lucene, the legacy name for Solr
- List key features of Solr
- Identify the advantages of Solr compared to Drupal search
Apache Solr is not a Drupal module, but a server application like Varnish or MySQL. Before we can use Solr with Drupal, we must plan how we will deploy Solr to our production site.
In this tutorial, we'll:
- List the requirements for Solr installation
- Identify when to install Solr on new hardware
- Describe various installation methods
By the end of this tutorial you should be able to describe a typical Solr install, and begin to list out the various things you'll need to do to install Solr for your environments.
Use Solr Locally
FreeJust as you would for Drupal, you should always test your search configuration prior to deploying it to production. In this tutorial, we examine the various ways to set up Apache Solr locally on your system. Then we'll walk through setting up DDEV with Solr for local development.
In this tutorial, we'll:
- Describe options for running Solr locally
- List which popular local development environments provide Solr
- Show how to set up a local, DDEV-based local dev environment with Solr
By the end of this tutorial you should be able to list the different ways that Solr can be installed locally, choose an option that works for you, and see how to get started quickly with DDEV.
Solr compartmentalizes itself into cores (or collections if you're using SolrCloud). Each Solr core has its own directory, configuration, and set of search data. While a core can be thought of as an “index”, it is much more.
In this tutorial, we'll:
- Identify the difference between a Solr core and an index.
- List the various ways a core can be created.
- Explain why Search API needs a custom core configuration.
By the end of this tutorial you should be able to explain what Solr cores are, and how to create a Solr core (or collection) that is compatible with Drupal's Search API module.
In order for Drupal to work with Apache Solr, we need to add the Search API module. This module provides a generic interface for search backends, including Solr. Furthermore, it adds several features to search without the need for custom code.
In this tutorial, we'll:
- Describe why Search API is necessary to use Solr with Drupal
- Identify a Search API server
By the end of this tutorial you should be able to install the Search API and Search API Solr modules, and create the Search API server configuration required to connect Drupal and Solr.
When developing a Drupal site, it is best practice to maintain multiple environments: A production environment for your live site, a stage environment for “next version” development, and your local environment for debugging and creating new features. Solr adds further complexity as we should have a separate Solr server for each.
In this tutorial, we'll:
- Describe why different Solr servers should be used for each environment
- Explain why Config Split is not a solution for multiple environments
- Describe how to use config overrides for each environment
By the end of this tutorial you should be able to override your Search API server configuration with environment-specific settings.
While Solr compartmentalizes settings into cores, Search API organizes things into indexes. Each Search API index can have a unique set of settings and crawl a specified list of content types. Search API indexes can be created in the Search API admin interface.
In this tutorial, we'll:
- Identify a Search API index
- Describe how an index is related to a Solr core
By the end of this tutorial you should be able to create a new Search API Index connected to a Solr backend.
Creating an index alone is not enough. To populate the index, we need to specify the fields necessary to populate the index. Selecting the fields is accomplished in the Search API admin UI.
In this tutorial, we'll:
- Explain how to select what populates a search index
- Describe a field boost, and how it is used to customize results
By the end of this tutorial you should be able to add fields to a Search API Index so their content is available for searching, and then instruct Search API to index the content of your site.
Reference field types, such as taxonomy term fields, paragraph fields, or plain entity reference fields, refer to a completely separate entity within the site. This makes search configuration complicated as the typical scope of a search crawl is on a per-node (really a per-entity) basis. Fortunately there are known strategies to index these fields with ease.
In this tutorial, we'll:
- Describe why reference types pose a particular challenge to indexing
- Discuss the importance of display modes in indexing
- Highlight how the Rendered HTML Output field can be used to index paragraphs
By the end of this tutorial you should be able to add reference fields to your Search API Index and allow users to search their contents in the correct context.
One of Search API’s key advantages is that custom search pages can be created using Views. This allows a high degree of customization, while relying on a familiar toolset.
In this tutorial, we'll:
- Describe how to use Views to create a search page
- Explain search page best practices, including requiring input and no-results text
By the end of this tutorial you should be able to create a page that users can use to search your site's content using the Solr search backend.
Drupal has the ability to support multiple Search API indexes within a single installation. While adding a new index is easy, we must understand the implications of creating and using multiple Search API indexes.
In this tutorial, we'll:
- Identify when to create multiple indexes in Search API
- Define virtual indexes, and their performance implications
Processors allow you to augment your search indexes by performing additional operations before or after the index operation. This can make your search more flexible.
In this tutorial, we'll:
- Identify what a processor is, and when it can be employed in the search pipeline.
- List useful processors provided by Search API.
- Describe how to apply a processor to an index, and why reindexing is necessary.