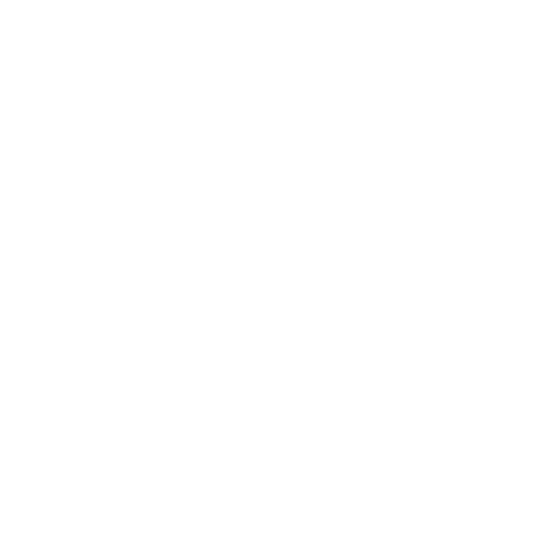
Add user data to the database from an existing form.
Display custom content using a render array.
Render content which can be refreshed via Ajax.
Create a custom block with details from the database containing information about user preferences.
Every Drupal module needs a *.info.yml file; the basic structure of a form controller class and related routing is the same for every form; and much of the code required to create a custom content entity type is boilerplate annotations and extending base classes. Wouldn't it be nice if there was a way to automate some of that repetitive work? Drush can be used to speed up module development by generating scaffolding code for event subscribers, forms, services, module files, routing, and much more. These generators are provided by the Drupal Code Generator project. They're neatly bundled up in Drush under the drush generate
command.
Before Drush 9, there were no code generators in Drush, but the Drupal Console project provided them. That project and its code generators, have languished since the release of Drupal 9. While it can still be used, and is often referenced in tutorials about Drupal, we much prefer the code generated by Drush at this point.
In this tutorial we'll:
- Learn about the Drupal Code Generator project
- Learn how Drush integrates with this project
- Demonstrate the
drush generate
command and its options
By the end of this tutorial, you'll know how to use the drush generate
command to speed up development for your Drupal modules.
A multisite Drupal installation allows you to host multiple, separate websites while relying on the same set of code. Large organizations often rely on a multisite installation to cut down on the operational upkeep of multiple sites. Hosting a multisite in Docker poses several additional challenges. Fortunately, the process is not dissimilar from configuring a bare-metal server to run a multisite.
In this tutorial, we'll:
- Outline a multisite's additional requirements for Docker containers.
- Configure alternate, local domain names to resolve each site.
- Learn how to configure a multisite to use alternate domain names.
This exercise will have you demonstrate knowledge of the installation process and how to customize Drupal for an improved experience when using the administration UI.
This exercise requires you to analyze wireframes detailing the architecture of a website and design a content type to store all the required information.
In this exercise you will demonstrate the ability to build a ‘Movie Review’ content type based on our design by downloading contributed modules to provide all the necessary field types.
This exercise will require you to build a view which lists films filtered by genre and create image styles to format images for different purposes.
In this exercise you will demonstrate more advanced Views skills by building a view with a relationship between content and taxonomy and formatting the output using a table.
In this exercise you will demonstrate knowledge of Panels, and create a layout for the Top Ten List page, and create and configure content within Panel Panes.
In this exercise you will build and position quicktabs within a panel page.
In this exercise you will create and position menus, static pages, and webforms, which can be accessed via the menu.
In this exercise you will combine knowledge of Panels and Views to create a complex page layout.
In this exercise you will create URL aliases for all content, and create user roles to allow logged-in users to create content.
In this exercise you will demonstrate knowledge of core search by enabling site search, configuring the search display to format the content, and indexing the content.
In this exercise you will demonstrate your ability to create a custom theme as a subtheme based on Zen and use Sass.
This exercise will have you demonstrate an understanding of Drupal themes and how to develop CSS to theme the default markup created by Drupal, with particular reference to CSS naming conventions.
Installing Drupal using the instructions in this tutorial will give you a working Drupal site that can be used for learning, or real-world project development.
Before you can work on a Drupal site locally (on your computer), you'll need to set up a local development environment. This includes all the system requirements like PHP and a web server, that Drupal needs in order to run. Our favorite way to accomplish this is using DDEV.
In this tutorial we'll learn:
- How to install and configure DDEV for use with a Drupal project.
- How to use DDEV's integrated Composer to download Drupal and Drush.
- How to install Drupal inside DDEV so you can access the site and start doing development.
By the end of this tutorial, you should be able to set up a local development environment for learning Drupal or working on a new Drupal project.